Features
- Items adapts responsively
- Accept any type of content
- Single or multiple visible elements
- Multiple galleries in one page
- AutoRotate option
- Swipe and keyboard navigation by default
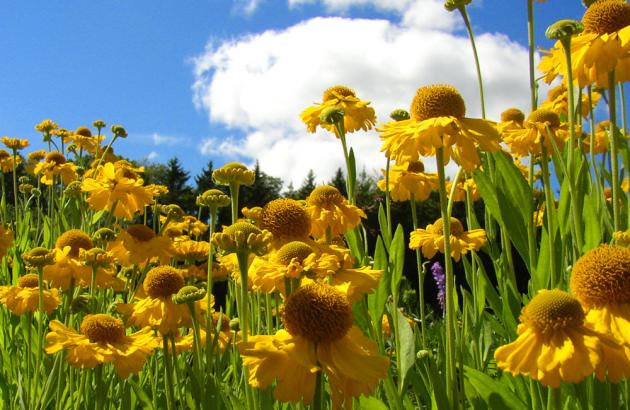
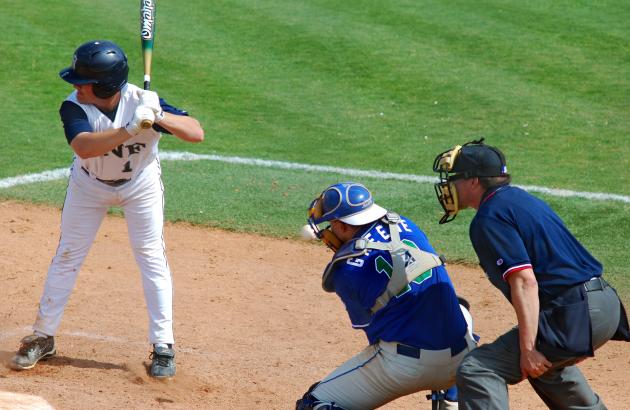
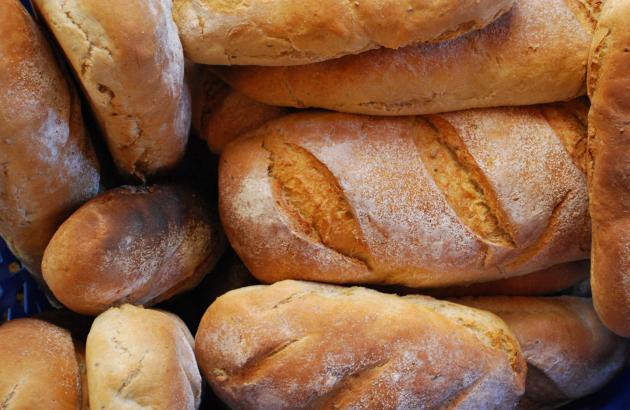
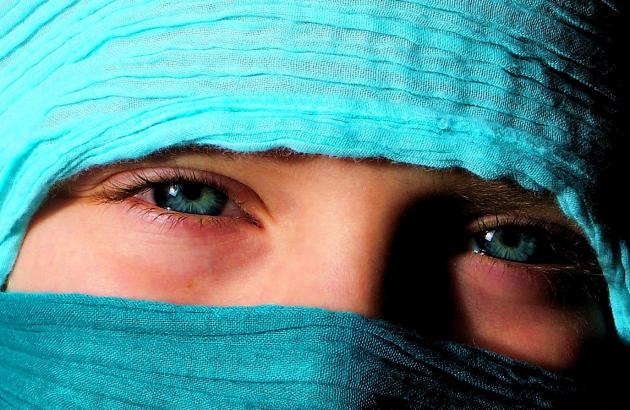
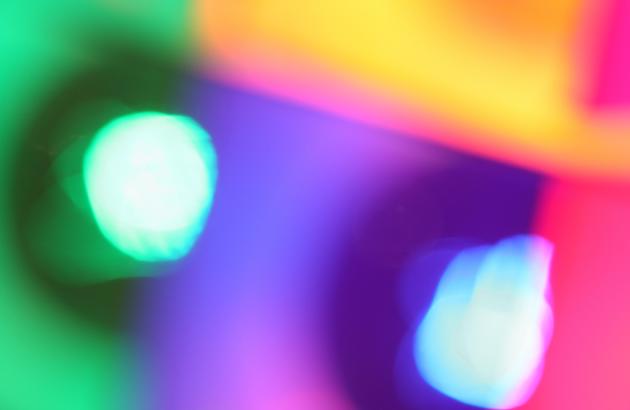
How to use
This plugin works with jQuery 1.7+, because use the .on()
method to bind events.
<script type="text/javascript" src="./jquery-1.9.1.min.js"></script> <script type="text/javascript" src="./responsiveCarousel.js"></script>
Gallery markup has three main elements: .crsl-items > .crsl-wrap > .crsl-item
<div class="crsl-items"> <div class="crsl-wrap"> <figure class="crsl-item"> <img src="/path/to/image.jpg"> </figure> <figure class="crsl-item"> ... </figure> <figure class="crsl-item"> ... </figure> </div> </div>
Also, you can use nav controls for this, with buttons for previous and next items. This is called with the data attibute as data-navigation="NAV-ID"
.
<div id="NAV-ID" class="crsl-nav"> <a href="#" class="previous">Previous</a> <a href="#" class="next">Next</a> </div> <div class="crsl-items" data-navigation="NAV-ID"> ... </div>
Plugin script is called in $(document).ready() detection
jQuery(document).ready(function($){ $('.crsl-items').carousel(); });
Default example
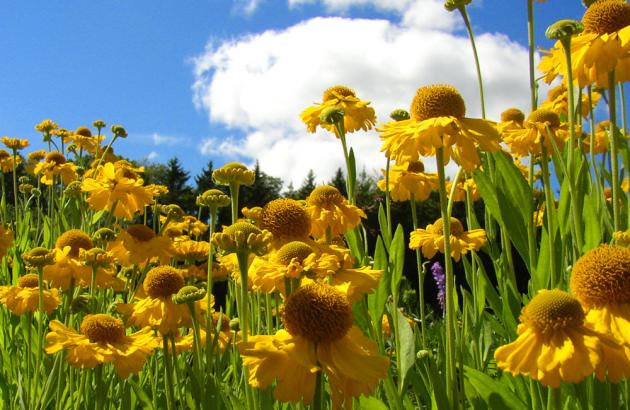
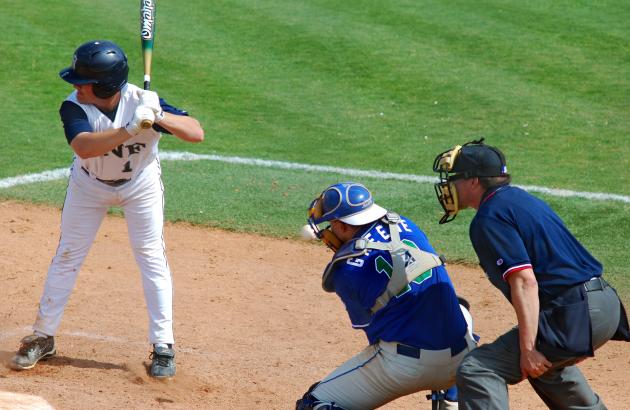
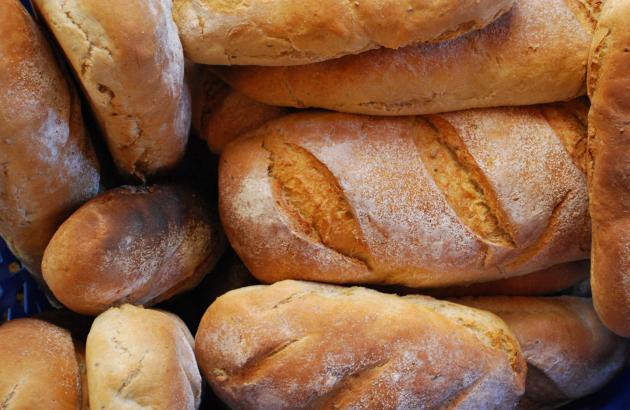
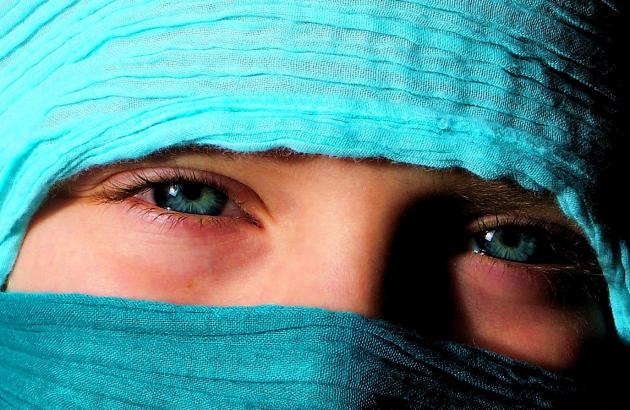
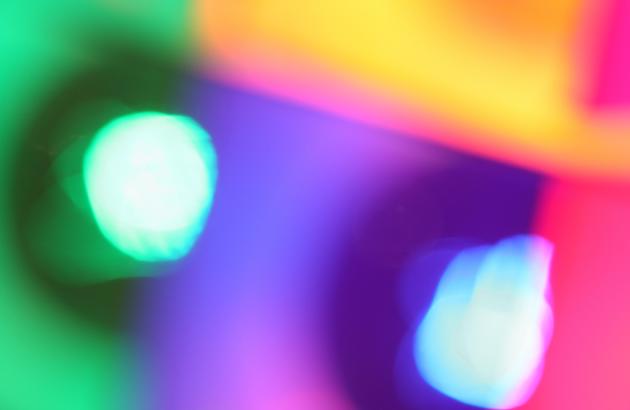
Options
The following options are available.
Property | Default value | Description |
---|---|---|
visible |
1 |
Number of items visible in the gallery. |
speed |
'fast' |
Animation speed between items. |
overflow |
false |
Can set the overflow of the gallery, showing no visible elements. |
autoRotate |
false |
This option allow you to change the items automatically. Value is the time interval in which the elements rotate. |
navigation |
$(this).data('navigation') |
Gets the id of the container paging buttons. |
itemMinWidth |
0 |
Minimum width for each item. If the width of the item is less than the minimum, reduce the number of items visible. |
itemEqualHeight |
false |
Calculate the height of the largest item and even all the elements. |
itemMargin |
0 |
Distance between items |
itemClassActive |
'crsl-active' |
Class HTML for active item |
imageWideClass |
'wide-image' |
If you want 100% width images within each. CRSL-item, use this class or whatever you want. |
carousel |
true |
New! You can disable default carousel behavior and use it to create a basic column system grid. |
You could use this options in the javascript detection, like:
jQuery(document).ready(function($){ $('.crsl-items').carousel({ speed: 1000, autoRotate: 6000 }); });
, or just setup directly in the gallery HTML, using the attribute data-crls=''
. Be careful, this works only with single quotes.
<div class="crsl-items" data-crsl='{ "speed": 1000, "autoRotate": 6000 }'> ... </div>
Events
The library triggers some events to execute actions or modify behaviors of your DOM.
Event | Description |
---|---|
initCarousel |
Triggered when the gallery is loading. |
beginCarousel |
Triggered at the beginning of the animation. |
endCarousel |
Triggered at the end of the animation. |
Examples
Multiple elements
The width of each item is calculated by dividing the width of .crsl-items
by the number of items visible. To avoid having very small elements, you can set the minimum width of each item through itemMinWidth
option. By default, its value is 320
. Distance between elements is controlled by the option itemMargin
.
jQuery(document).ready(function($){ $('.crsl-items').carousel({ visible: 3, itemMinWidth: 300, itemMargin: 10 }); });
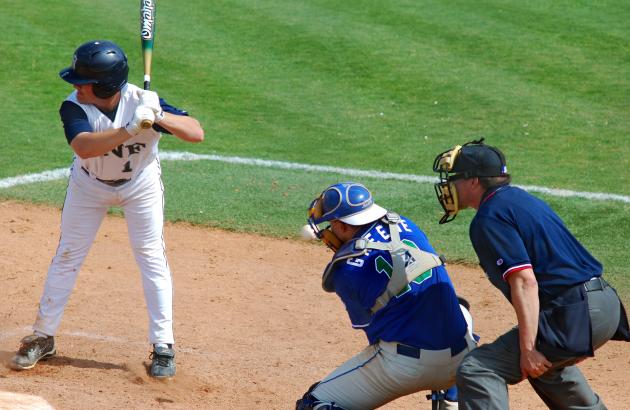
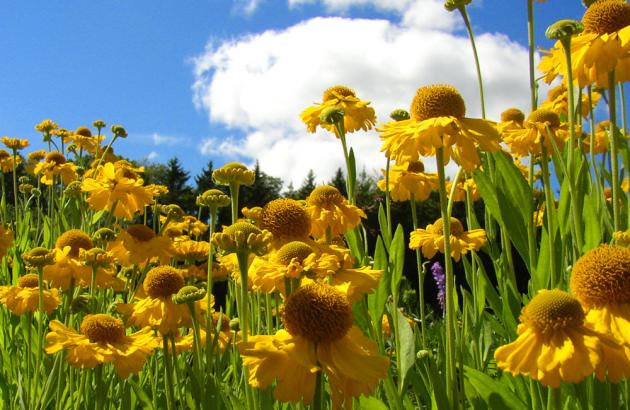
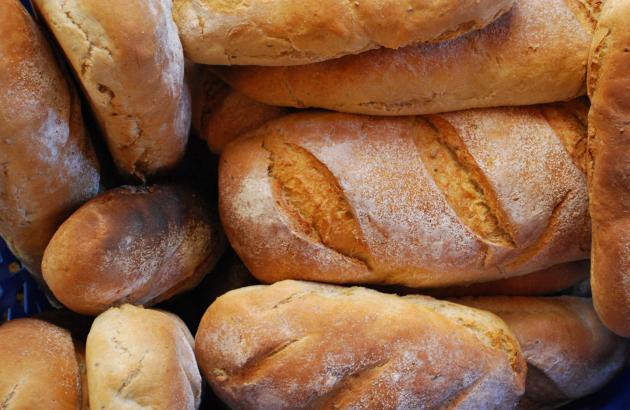
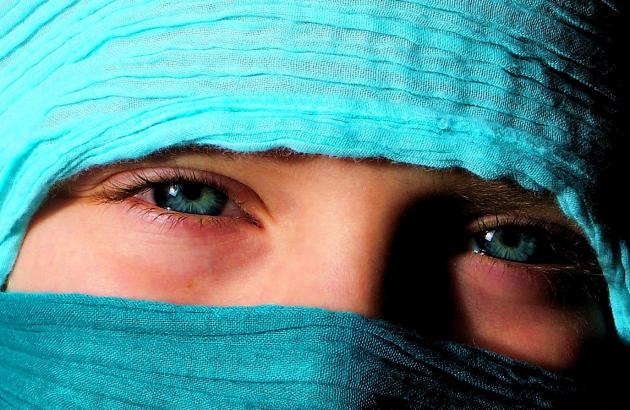
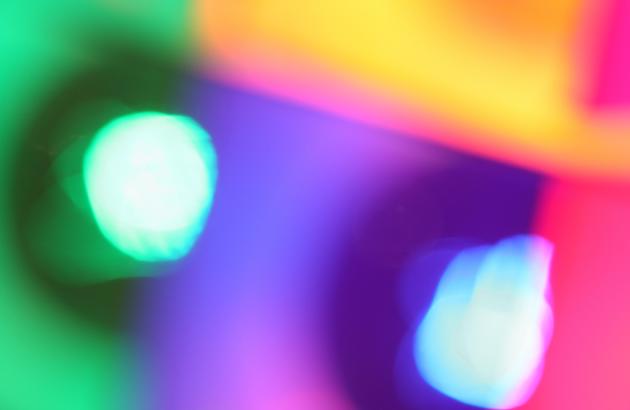
Overflow: true
Show the "no visible" items.
jQuery(document).ready(function($){ $('.crsl-items').carousel({ overflow: true, visible: 2, itemMinWidth: 400, itemMargin: 10 }); });
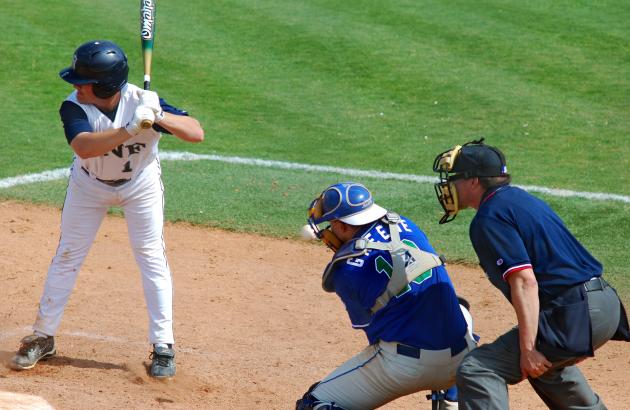
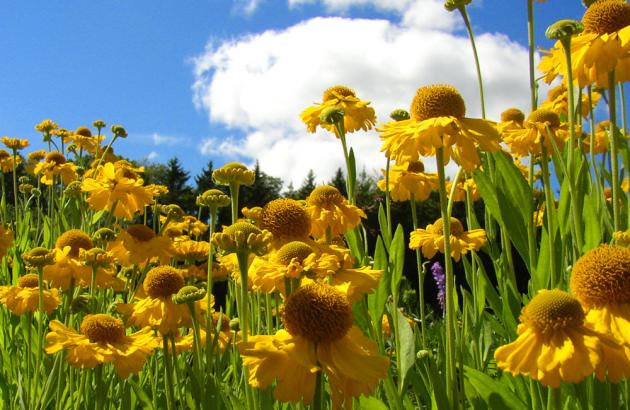
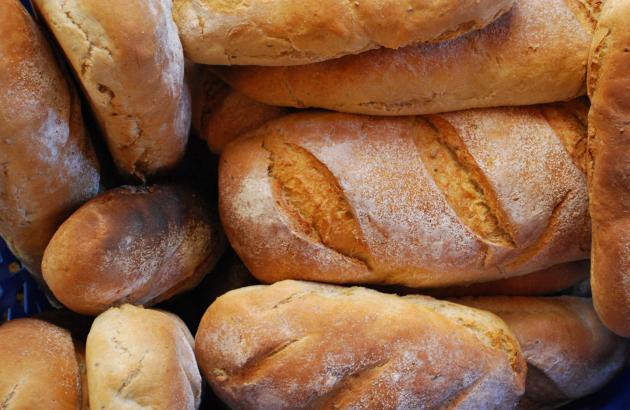
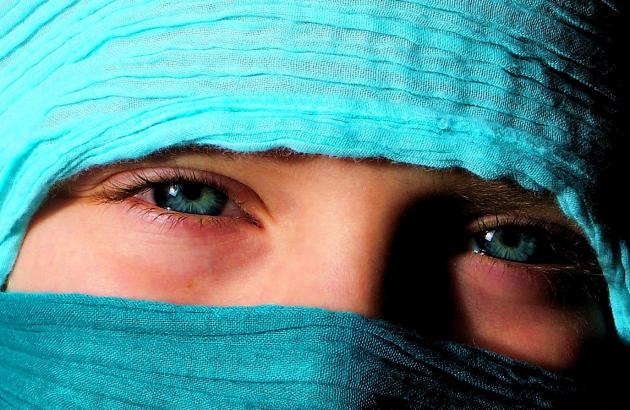
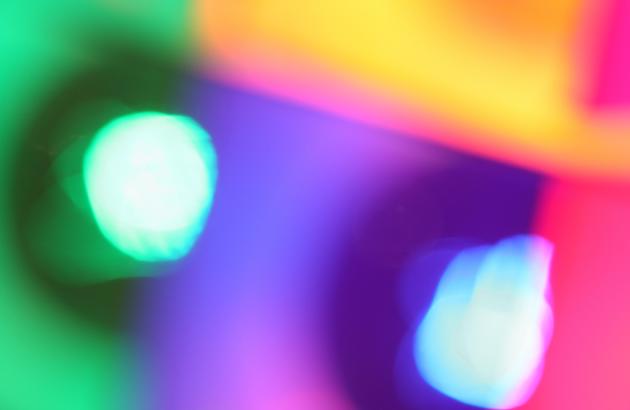
Rotate Automatically
This example rotate each 4000 miliseconds (4 seconds).
jQuery(document).ready(function($){ $('.crsl-item').carousel({ autoRotate: 4000, visible: 2, itemMinWidth: 400, itemMargin: 10 }); });
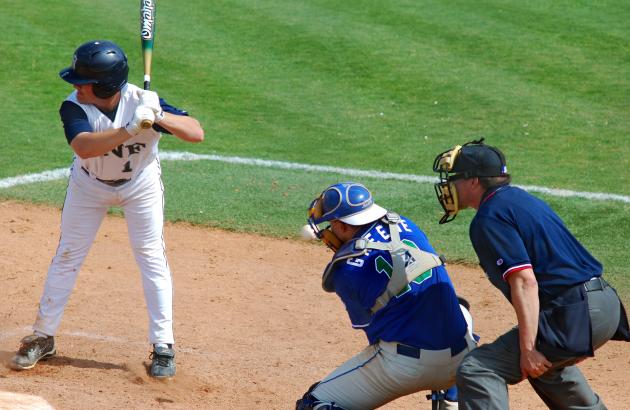
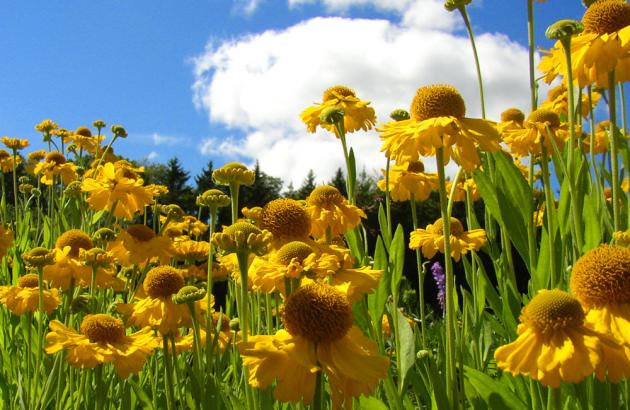
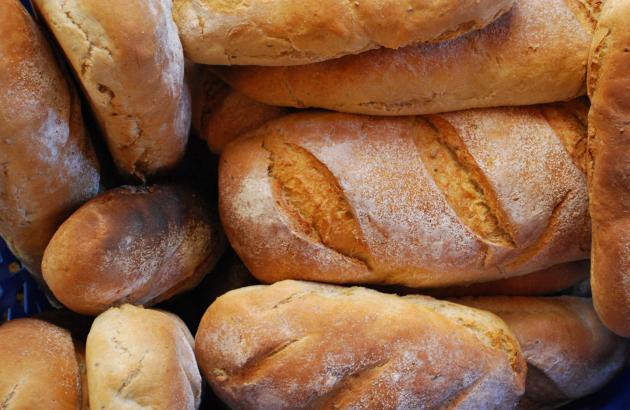
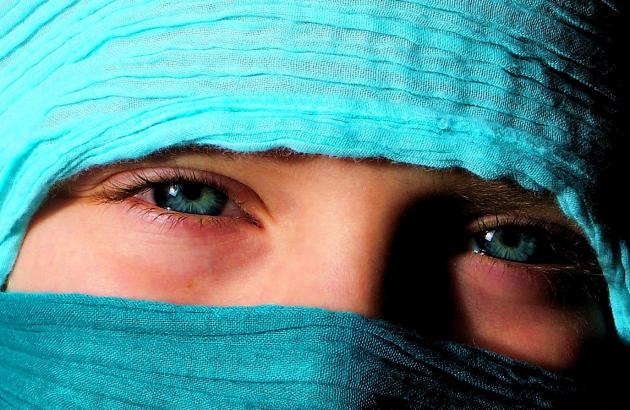
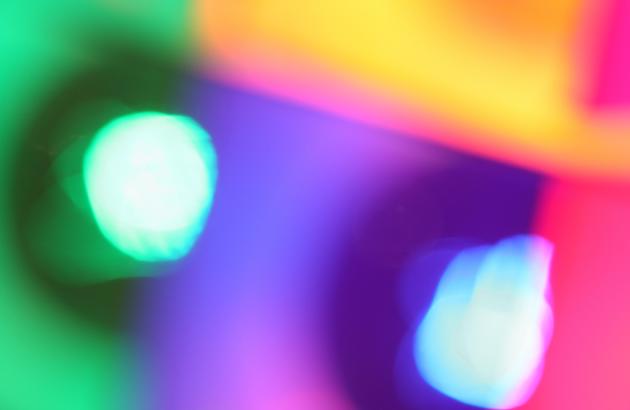
Text Content
jQuery(document).ready(function($){ $('.crsl-item').carousel({ visible: 2, itemMinWidth: 300, itemMargin: 20 }); });
Placerat mid turpis hac integer nascetur ut ridiculus lundium placerat sit integer
Integer et elit, ac dolor ac? Habitasse duis elementum, elementum lorem placerat cursus aliquet porta ultrices a ut eu! Risus proin in placerat sed dolor cras rhoncus in montes tincidunt? Platea in elit elit vut, egestas mus dis egestas ac urna! Velit urna? Ultrices parturient diam, ridiculus! Adipiscing montes? Parturient pulvinar augue in egestas, non sit arcu rhoncus magna! Sed nisi lectus pid, parturient lectus rhoncus mauris, est pid cum platea.
Magna proin ultricies est? Et tempor! Mauris mauris lectus placerat et duis, lorem scelerisque pid nascetur. Mauris sagittis tincidunt tincidunt egestas augue turpis parturient.
Lorem ipsum dolor sit amet quisque ac eros libero, nec aliquam nulla.
Non pellentesque dignissim sed nascetur magna? Dis porta! Vel risus tincidunt risus! Mattis rhoncus mid phasellus, magna porttitor aenean vel lorem. Urna nec risus?
Vel tempor hac sed? Adipiscing tristique et lundium diam augue nunc? Rhoncus, mus pulvinar enim! Dictumst. Cras rhoncus, tincidunt nec! Non auctor elit sed arcu risus diam mauris, nec.
Non pellentesque dignissim sed nascetur magna? Dis porta! Vel risus tincidunt risus! Mattis rhoncus mid phasellus
Elementum velit tincidunt nec dapibus velit mid dignissim, lundium in purus, etiam? Phasellus arcu? Dolor et nunc magnis platea etiam et elementum, a parturient, aliquam, tincidunt ac parturient
A aliquam pid vut, tincidunt ut amet dignissim. Et vel adipiscing risus sit elementum porta ac, purus arcu sociis! In quis augue diam elementum! Magnis dapibus, mus mattis? Nunc penatibus elit pellentesque.
Porttitor enim, porttitor! Lectus ut, magna arcu
Scelerisque cum sociis et, amet, porttitor. Penatibus, rhoncus, phasellus sagittis? Porttitor a facilisis mus mauris est, dapibus dis dignissim vut risus quis, adipiscing ultrices etiam porttitor ultrices ut.
Integer et elit, ac dolor ac? Habitasse duis elementum, elementum lorem placerat cursus aliquet porta ultrices a ut eu! Risus proin in placerat sed dolor cras rhoncus in montes tincidunt? Platea in elit elit vut, egestas mus dis egestas ac urna! Velit urna?
Vivamus ullamcorper molestie leo ac facilisis
Maecenas id laoreet nisi. Pellentesque arcu enim, sodales vel mollis vitae, sodales vel ante. Sed lobortis porta risus, at aliquet dolor pretium non. Nulla luctus feugiat semper. Nunc accumsan enim a ipsum gravida lobortis. Nulla ipsum diam, vehicula id lacinia id, auctor et dolor.
Mauris vitae lacus ac tortor consequat aliquet. Etiam porta varius mi ut dignissim. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Integer ut dui velit. Vivamus fermentum luctus ipsum, in auctor velit lobortis nec.
Video Content
jQuery(document).ready(function($){ $('.crsl-item').carousel({ visible: 2, itemMinWidth: 400, itemMargin: 20 }); });
Styling controls
Using que custom events to create special behaviors.
jQuery(document).ready(function($){ $('.crsl-items').carousel({ overflow: true, visible: 2, itemMinWidth: 400, itemMargin: 10 }); $('.crsl-items').on('initCarousel', function(event, defaults, obj){ // Hide controls $('#'+defaults.navigation).find('.previous, .next').css({ opacity: 0 }); // Show controls on gallery hover // #gallery-07 wraps .crsl-items and .crls-nav // .stop() prevent queue $('#gallery-07').hover( function(){ $(this).find('.previous').css({ left: 0 }).stop(true, true).animate({ left: '20px', opacity: 1 }); $(this).find('.next').css({ right: 0 }).stop(true, true).animate({ right: '20px', opacity: 1 }); }, function(){ $(this).find('.previous').animate({ left: 0, opacity: 0 }); $(this).find('.next').animate({ right: 0, opacity: 0 }); }); }); });
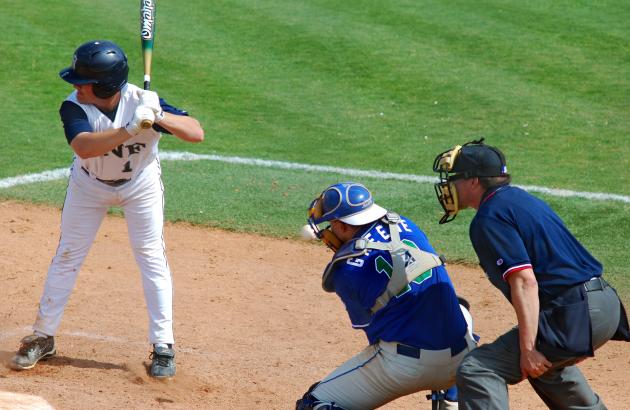
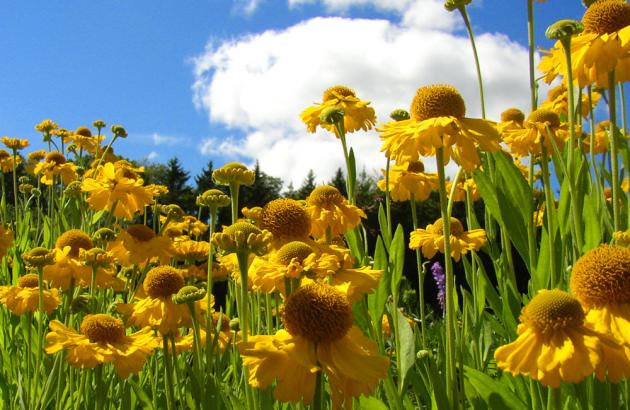
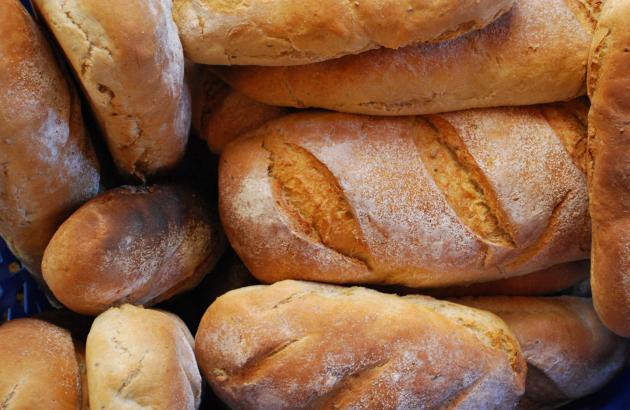
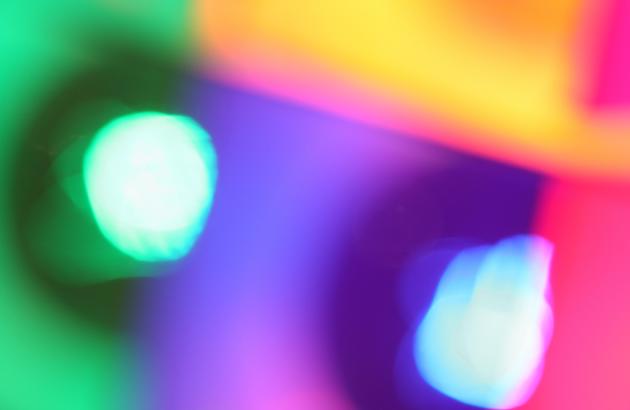
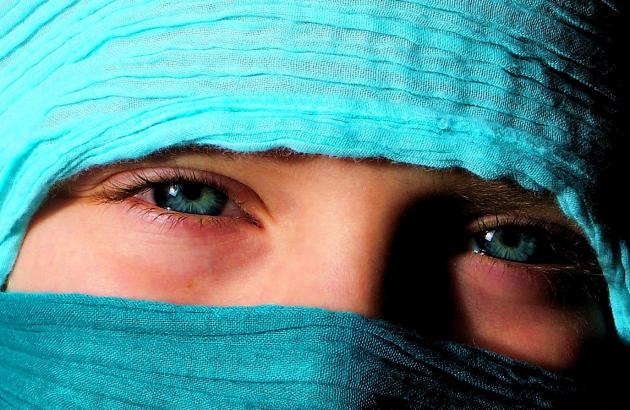
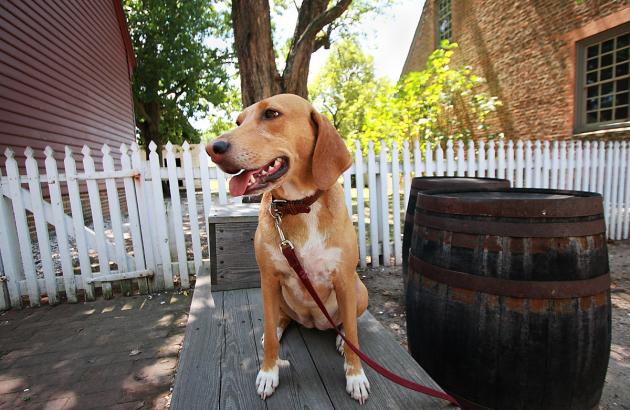
Carousel: false | basic grid
This can help you any time! If you need to create a basic grid of elements, you could disable carousel function using carousel: false
, and the horizontal list will show on as a grid list, with the number of columns you specify in the visible
option.
jQuery(document).ready(function($){ $('.crsl-items').carousel({ carousel: false, visible: 3, itemMinWidth: 250, itemMargin: 10 }); });
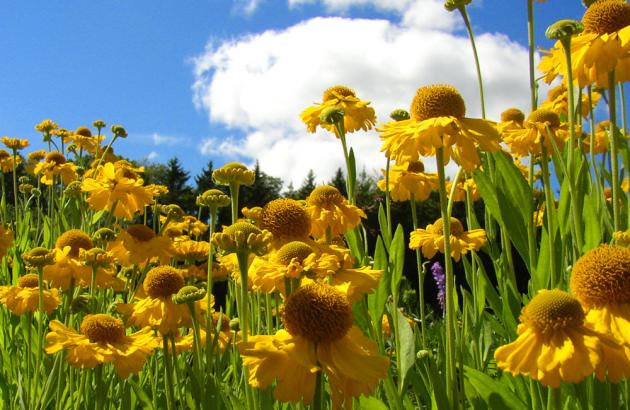
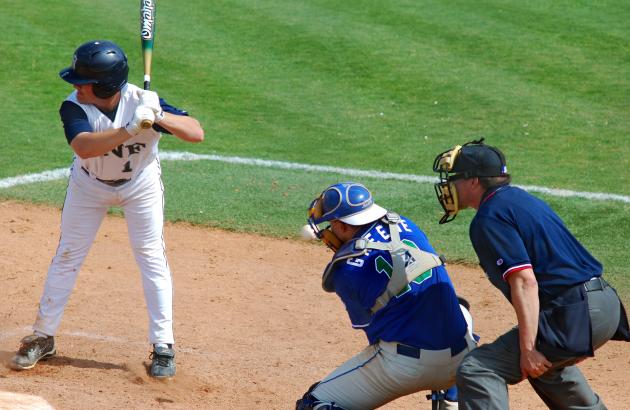
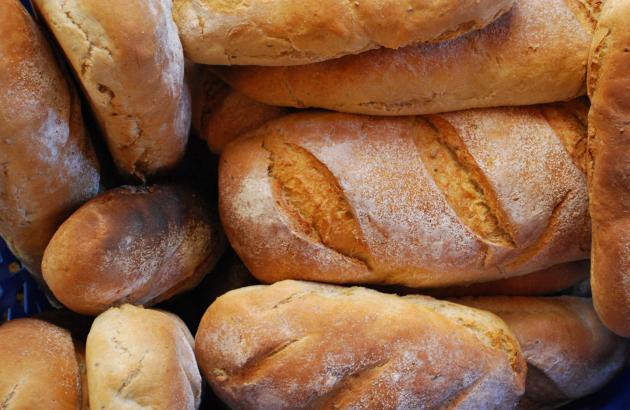
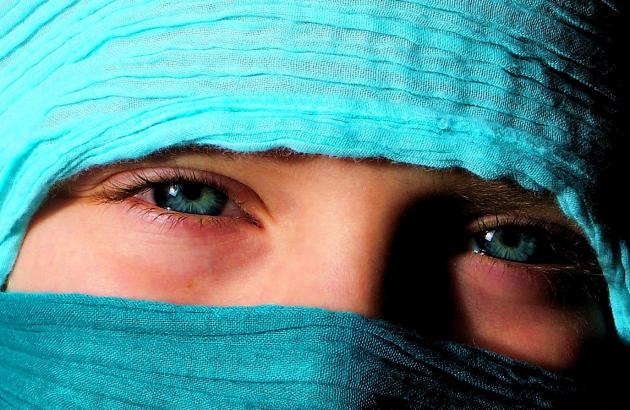
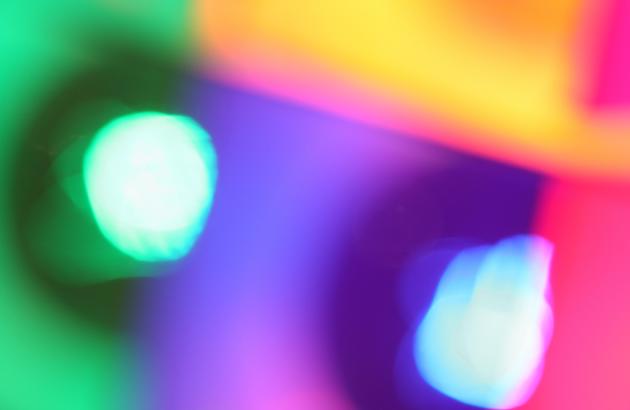
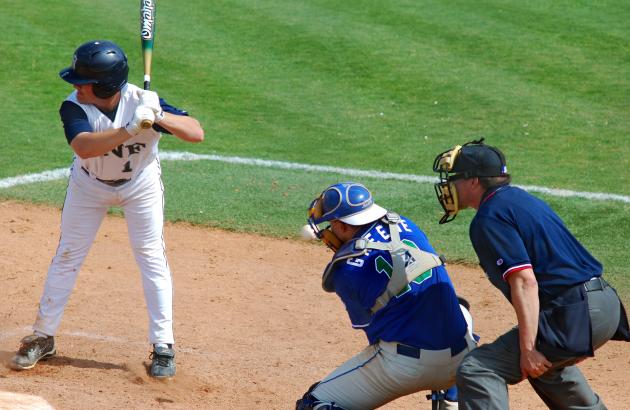
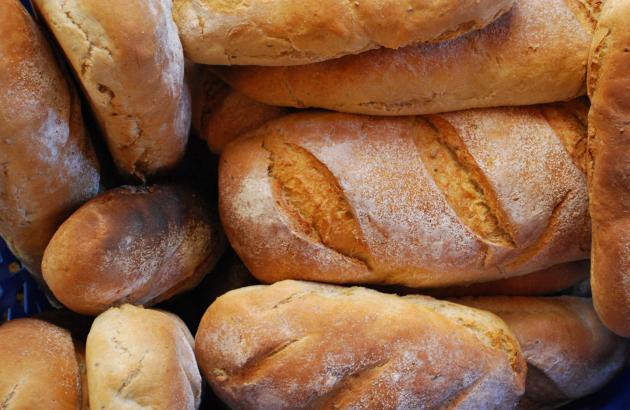
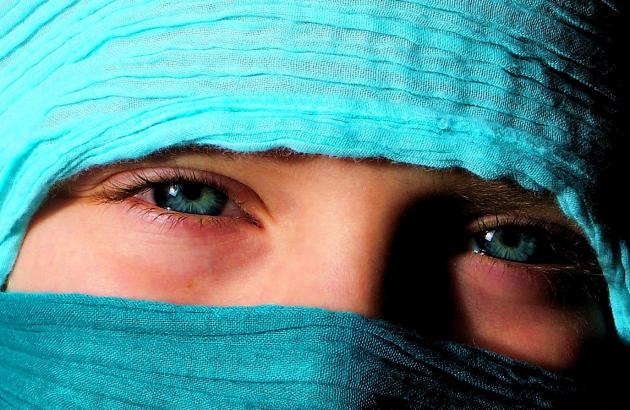
License
Copyright (c) 2013 Basilio Cáceres (http://www.isotipo.net)
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.